You are viewing an older version of the site. Click here to view
the latest version of this page. (This may be a dead link, if so, try the root page of the docs
here.)
== register_command ==
[[../objects/ms.lang.NotFoundException|ms.lang.NotFoundException]] |- ! scope="row" | Since | 3.3.1 |- ! scope="row" | Restricted |
Copy Code
The output might be:
Registers a command to the server's command list, or updates an existing one. Options is an associative array that can have the following keys: description, usage, permission, noPermMsg, aliases, tabcompleter, and/or executor.
=== Vital Info ===
{| style="width: 40%;" cellspacing="1" cellpadding="1" border="1" class="wikitable"
|-
! scope="col" width="20%" |
! scope="col" width="80%" |
|-
! scope="row" | Name
| register_command
|-
! scope="row" | Returns
| boolean
|-
! scope="row" | Usages
| commandname, optionsArray
|-
! scope="row" | Throws
| [[../objects/ms.lang.FormatException|ms.lang.FormatException]][[../objects/ms.lang.NotFoundException|ms.lang.NotFoundException]] |- ! scope="row" | Since | 3.3.1 |- ! scope="row" | Restricted |
Yes
|-
! scope="row" | Optimizations
| None
|}The 'noPermMsg' argument is the message displayed when the user doesn't have the permission specified in 'permission' (unused as of Spigot 1.19). The 'usage' is the message shown when the 'executor' returns false. The 'executor' is the closure run when the command is executed, and can return true or false (by default is treated as true). The 'tabcompleter' is the closure run when a user hits tab while the command is entered and ready for args. It is meant to return an array of completions, but if not the tab_complete_command event will be fired, and the completions of that event will be sent to the user. Both executor and tabcompleter closures are passed the following information in this order: alias used, name of the sender, array of arguments used, array of command info.
When this function is not supported on the used platform, a NotFoundException is thrown. When the command name contains whitespaces, a FormatException is thrown.
In most simple cases, the tabcompleter can be easily created using the {{function|get_tabcompleter_prototype}} function, though for complex completion scenarios, you may prefer writing a custom closure anyways.
=== Usages ===
register_command(commandname, optionsArray)=== Examples === ====Example 1==== Register the /hug <player> command. Given the following code:
register_command('hug', array(
'description': 'Spread the love!',
'usage': '/hug <player>',
'permission': 'perms.hugs',
'tabcompleter':
closure(@alias, @sender, @args) {
// This replicates the default tabcompleter for registered commands.
// If no tabcompleter is set, this behavior is used.
@input = @args[-1];
return(array_filter(all_players(), closure(@key, @value) {
return(length(@input) <= length(@value)
&& equals_ic(@input, substr(@value, 0, length(@input))));
}));
},
'aliases': array('hugg', 'hugs'),
'executor':
closure(@alias, @sender, @args) {
if(array_size(@args) == 1) {
@target = @args[0];
if(ponline(@target)) {
broadcast(colorize('&4'.@sender.' &6hugs &4'.@target));
} else {
msg(colorize('&cThe given player is not online.'));
}
return(true);
}
return(false); // prints usage
}
));
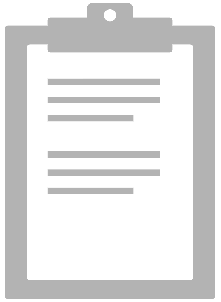
01 {{function|register_command}}('hug', {{function|array}}(
02 'description': 'Spread the love!',
03 'usage': '/hug <player>',
04 'permission': 'perms.hugs',
05 'tabcompleter':
06 {{keyword|closure}}(@alias, @sender, @args) {
07 // This replicates the default tabcompleter for registered commands.
08
09 // If no tabcompleter is set, this behavior is used.
10
11 @input = @args[-1];
12 {{function|return}}({{function|array_filter}}({{function|all_players}}(), {{keyword|closure}}(@key, @value) {
13 {{function|return}}({{function|length}}(@input) <= {{function|length}}(@value)
14 && {{function|equals_ic}}(@input, {{function|substr}}(@value, 0, {{function|length}}(@input))));
15 }));
16 },
17 'aliases': {{function|array}}('hugg', 'hugs'),
18 'executor':
19 {{keyword|closure}}(@alias, @sender, @args) {
20 {{keyword|if}}({{function|array_size}}(@args) == 1) {
21 @target = @args[0];
22 {{keyword|if}}({{function|ponline}}(@target)) {
23 {{function|broadcast}}({{function|colorize}}('&4'.@sender.' &6hugs &4'.@target));
24 } {{keyword|else}} {
25 {{function|msg}}({{function|colorize}}('&cThe given player is not online.'));
26 }
27 {{function|return}}({{keyword|true}});
28 }
29 {{function|return}}({{keyword|false}}); // prints usage
30
31 }
32 ));
Registers the /hug command.===See Also===
[[API/functions/set_tabcompleter.html|set_tabcompleter]]
, [[API/functions/set_executor.html|set_executor]]
, [[API/functions/unregister_command.html|unregister_command]]
, [[API/functions/get_tabcomplete_prototype.html|get_tabcomplete_prototype]]
Find a bug in this page? Edit this page yourself, then submit a pull request. (Note this page is automatically generated from the documentation in the source code.)